Save to My DOJO
Table of contents
In my last article, I left you with some PowerShell technique for getting Hyper-V performance counters from Windows Management Instrumentation (WMI) using Get-CimInstance. I’ll admit that getting the information from WMI is bit advanced. But PowerShell has another cmdlet called Get-Counter that might be able to simplify the process. Although I expect you’ll want to massage this output as well. Don’t worry. I’ve got plenty of code examples to get you started.
The code I’m going to show you should work against a remote server. Although as a last resort you could run my commands in a remote session using Invoke-Command. To make things easy for you to follow along, I’m going to set a variable for the computer I want to query, just as I did in previous articles. In this case, this will be my Windows 10 laptop running Hyper-V.
$computer = $env:COMPUTERNAME
The Get-Counter cmdlet can list all available counter sets. For our purposes naturally, we are interested in Hyper-V counters.
How to use Get-Counter cmdlet
get-counter -listset 'Hyper-V*' -ComputerName $computer
In my setup, I am always most concerned about memory usage since that is the one resource that is always limited for me. So I only care about dynamic memory
The key bit of information is the list of counters. These are the things I can get data on. Here’s the really cool part. I can pipe this output back to Get-Counter and get results for all available counters and instances.
$m = get-counter -listset 'Hyper-V Dynamic Memory VM' -ComputerName $computer | get-counter
I saved the results to a variable so I can re-use it.
The output of Get-Counter is great to look at but a bit cumbersome. The CounterSamples property lists the counter and value. In the value, you can see the computername, (YPJH10) and the virtual machine name like SRV1 and DOM1. You may prefer a better look at this data.
$m.CounterSamples | sort InstanceName,Path | format-table -GroupBy InstanceName -property Path,Cookedvalue,timestamp
Here I’ve created a formatted table grouped by each instance name.
The Path values are a bit redundant so I’ll clean them up a bit with the help of a regular expression.
[regex]$rx = "(?<=\\).*(?=\)" $m.CounterSamples | sort InstanceName,Path | format-table -GroupBy InstanceName -property @{Name="Counter";Expression={Split-path $_.path -Leaf}}, Cookedvalue,timestamp,@{Name="VMHost";Expression={$rx.Match((Split-Path $_.path)).value.ToUpper()}}
( can get the counter itself by splitting the path to get the last part. The regular expression pulls out the Hyper-V hostname from between the slashes.
This is a bit prettier and easier to read, but I can’t do much with it other than sending to a file or printer. But since performance counters are about reporting anyway that doesn’t matter a great deal. In fact, I know how much you all love HTML reports so here’s something you can use to create another version of this information.
#requires -version 5.0 #requires -runasAdministrator #HVDynamicMemoryReport.ps1 [cmdletbinding()] Param( [Parameter(Position = 0, HelpMessage = "Enter the name of the Hyper-V Host")] [ValidateNotNullOrEmpty()] [string]$Computername = $env:COMPUTERNAME, [Parameter(HelpMessage = "Enter the filename for the html report")] [ValidateNotNullOrEmpty()] [ValidateScript( {Test-Path (Split-Path $_)})] [string]$Path = "$env:tempdynamicmemoryreport.htm" ) #a meta data value used in the html footer $scriptVersion = "1.1" [regex]$rx = "(?<=\\).*(?=\)" $Counters = get-counter -listset 'Hyper-V Dynamic Memory VM' -ComputerName $computername | get-counter $data = $counters.CounterSamples | Sort-Object -property InstanceName, Path | Group-Object -Property InstanceName $fragments = @("<H1>Dynamic Memory Performance</H1>") $fragments += "<H2>Hyper-V Host: $($Computername.toUpper())</h2>" foreach ($item in $data) { $fragments += "<h3>$($item.name.toupper())</h3>" $fragments += $item.group | Select-object -Property @{Name = "Counter"; Expression = {Split-path $_.path -Leaf}}, Cookedvalue, Timestamp | Sort-Object -Property Counter | ConvertTo-Html -Fragment -as Table } #define a here string for the html header with embedded style sheet $head = @" <title>Dynamic Memory Performance $($computername.toupper())</title> <style> body { background-color:#FAFAFA; font-family:Arial; font-size:12pt; } td, th { border:1px solid black; border-collapse:collapse; } th { color:white; background-color:black; } table, tr, td, th { padding: 0; margin: 0} tr:nth-child(odd) { background-color: lightgray } table { margin-left:50px; } img { float:left; margin: 0px 25px; } .footer { font-size:8pt; } .footer tr:nth-child(odd) {background-color: white} .footer td,tr { border-collapse:collapse; border:none; } </style> "@ #HTML to display at the end of the report [xml]$meta = [pscustomobject]@{ Date = Get-Date Author = "$env:USERDOMAIN$env:username" Script = $($myinvocation.invocationname) Version = $scriptVersion Source = $($Env:COMPUTERNAME) } | ConvertTo-Html -Fragment -as List $class = $meta.CreateAttribute("class") $meta.table.SetAttribute("class","footer") $footer = @" <br> <i> $($meta.innerxml) </i> "@ #create the HTML document ConvertTo-HTML -Head $head -Body $fragments -PostContent $footer | Out-File -FilePath $path -Encoding ascii Write-Host "Report saved to $path" -ForegroundColor Green
This script is designed to report on the Hyper-V dynamic memory counters, but you could modify it for probably any counter set. You could also modify it to limit reporting to a specific virtual machine by inserting some code to filter on the Instance. This script includes a CSS style sheet embedded into the report that will display table rows in alternate colors.
I think by now your head is spinning with PowerShell code so I’ll wrap it up for now so you have some time to try these things out. I hope you are finding these excursions into performance counters with PowerShell useful because I have a few more things in mind to share with you. In the meantime kick the samples around, in a test environment of course, and let me know what you think in the comments section below!
Want to learn more neat tips using PowerShell? Check out these 5 PowerShell Hacks for Hyper-V!
Are you having trouble getting your PowerShell scripts to run properly? Find out Why Your Hyper-V PowerShell Commands Don’t Work (and how to fix them)
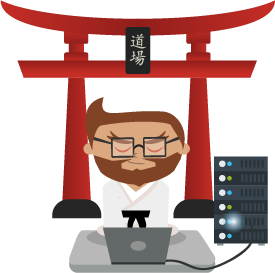
Not a DOJO Member yet?
Join thousands of other IT pros and receive a weekly roundup email with the latest content & updates!